The third type of iteration, which we shall use when the number of iterations is known in advance, is a for loop. This, in its simplest form, uses an initialization of the variable as a starting point, a stop condition depending on the value of the variable. The variable is incremented on each iteration until it reaches the required value.
The syntax is;
FOR (starting state, stopping condition, increment)
Statements
ENDFOR
Example 1:
FOR (n = 1, n <= 4, n + 1)
DISPLAY “loop”, n
ENDFOR
The fragment of code will produce the output
Loop 1
Loop 2
Loop 3
Loop 4
In the example, n is usually referred as the loop variable, or counting variable, or index of the loop. The loop variable can be used in any statement of the loop. The variable should not be assigned a new value within the loop, which may change the behavior of the loop.
Example 2: Write a program to calculate the sum and average of a series of numbers.
The pseudo-code solution is;
Use variables: n, count of the type integer
Sum, number, average of the type real
DISPLAY “How many numbers do you want to input”
ACCEPT count
SUM = 0
FOR (n = 1, n <= count, n + 1)
DISPLAY “Input the number from your list”
ACCEPT number
SUM = sum + number
ENDFOR
Average = sum / count
DISPLAY “The sum of the numbers is “, sum
DISPLAY “Average of the numbers is “, average
Flowcharts have been used in this section to illustrate the nature of the three control structures. These three are the basic control structures out of which all programs are built. Beyond this, flowcharts serve the programmer in two distinct ways: as problem solving tools and as tools for documenting a program.
Example 3: Design an algorithm and the corresponding flowchart for finding the sum of n numbers.
Pseudocode Program
Start
Sum = 0
Sum = 0
Display “Input value n”
Input n
For(I = 1, n, 5)
Input a value
Sum = sum + value
ENDFOR
Output sum
Output sum
Stop
In this example, we have used I to allow us to count the numbers, which we get for the addition. We compare I with n to check whether we have exhausted the numbers or not in order to stop the computation of the sum (or to stop the iteration structure). In such a case, I is referred to as a counter.
The corresponding flowchart will be as follows:
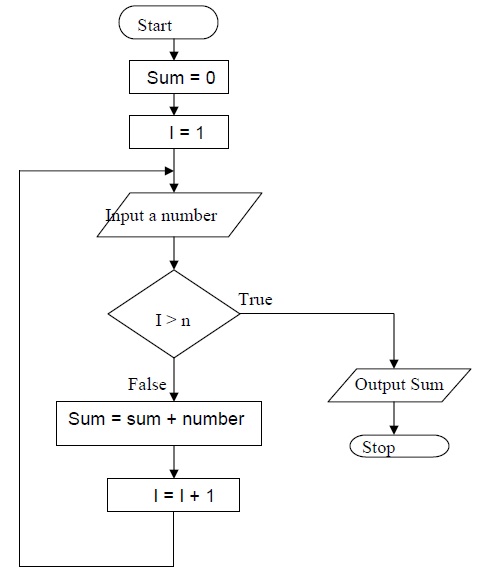